Kitchen Temperature Monitoring
Adding the DHT22 Sensor
So as per my doorbell post, I mentioned I would be adding a temperature and humidity sensor as well. Since my doorbell unit sits on a shelf in the Kitchen I thought it would be great to be able to monitor the ambient temperature and relative humidity in the kitchen. To input these variables into an infuxDB and then plot the data points on a graph using Grafana. This could be cool to see how the kitchen temperature and humidity increases during cooking times and during the day. Its also cool to do this because well... why not. :)
So I added a DHT22 sensor to the NodeMCU board that drives the now smart(ish) doorbell. I opted for the DHT22 over the DHT11 as it is more accurate and has a wider reading rating.
This is the spec I grabbed off HowToMechatronics site so I dont need to retype it. Also its a really cool site - check it out. He does great fun projects.
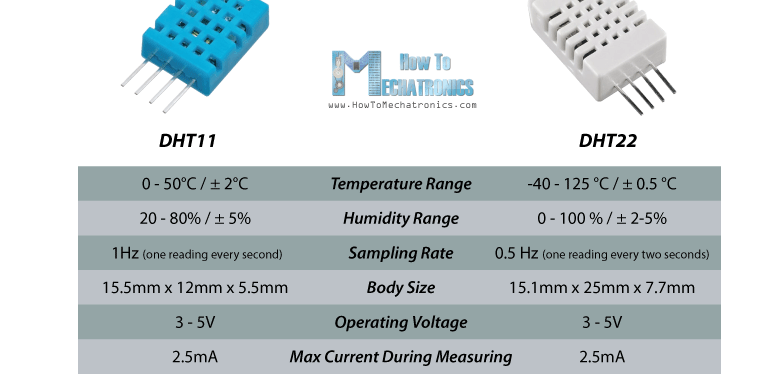
I grab a reading from the sensor every 50 seconds and publish the data over my MQTT network every 60 seconds. No particular reason for these values, Its just want I went with.
The code for handle this looks like this. The Serial.print is just for my debugging in the console, but do not actually get used in the running of the sketch.
void checkDHT() {
temperature = dht.readTemperature();
delay(2100);
humidity = dht.readHumidity();
if (isnan(humidity) || isnan(temperature)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
Serial.print("Sample OK: ");
Serial.print(temperature);
Serial.print(" *C, ");
Serial.print(humidity);
Serial.println(" RH%");
}
void publishDHT() {
client.publish(temperature_topic, String(temperature).c_str());
client.publish(humidity_topic, String(humidity).c_str());
}
Next I needed to add Number fields to my OpenHAB system so that I can see these temperatures from the app or web, as well as to eventually record them in influx and plot on Grafana (Still need to do this.)
Here are some images of the final product and data pulling through to the app.
Quick and easy, fun little task.
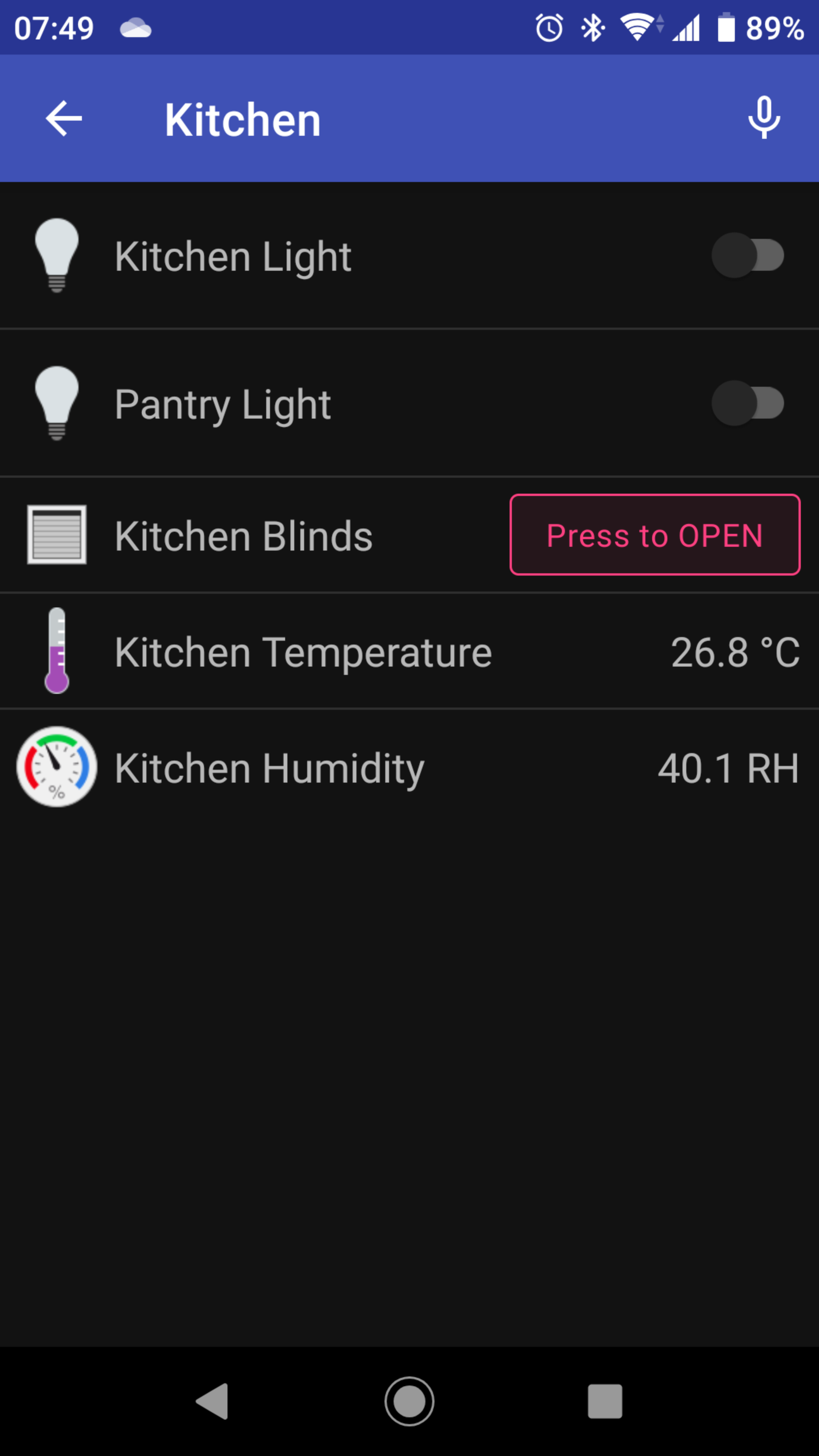